装饰者模式
设计模式
装饰( Decorator)模式又叫包装模式。通过一种对客户端透明的方式来扩展对象的功能,是继承关系的一个替换方案。
装饰模式就是把要添加的附加功能分别放在单独的类中,并让这个类包含它要装饰的对象,当需要执行时,容户端就可以有选择地、按顺序地使用装饰功能包装对象。
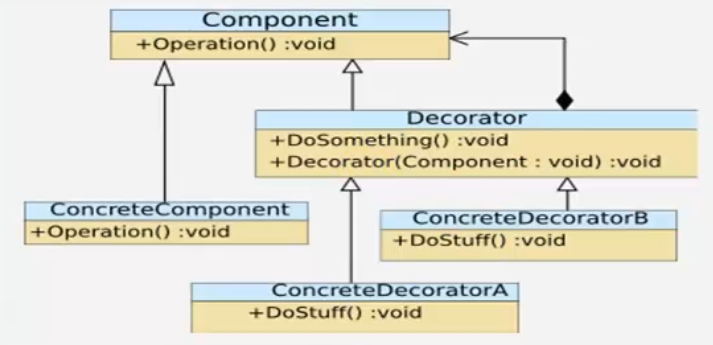
适用于:
装饰者模式( Decorator Pattern)动态的给一个对象添加一些额外的职责。就增加功能来说,此模式比生成子类更为灵活。
代码
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87
| #include <iostream>
using namespace std;
class Car { public: virtual void show() = 0; };
class RunCar : public Car { public: virtual void show() { cout << "可以跑。" << endl; } };
class SwimCarDirector : public Car { public: SwimCarDirector(Car* car) { m_car = car; } void swim() { cout << "可以游。" << endl; } virtual void show() { m_car->show(); swim(); } protected: private: Car* m_car; };
class FlyCarDirector : public Car { public: FlyCarDirector(Car* car) { m_car = car; } void fly() { cout << "可以飞。" << endl; } virtual void show() { m_car->show(); fly(); } protected: private: Car* m_car; };
int main() { Car* myCar = new RunCar; myCar->show();
cout << "--------------" << endl;
FlyCarDirector* flyCar = new FlyCarDirector(myCar); flyCar->show();
cout << "--------------" << endl;
SwimCarDirector* swimCar = new SwimCarDirector(myCar); swimCar->show();
cout << "--------------" << endl;
SwimCarDirector* swimCar2 = new SwimCarDirector(flyCar); swimCar2->show();
cout << "--------------" << endl;
cout << "Hello world!" << endl; return 0; }
|
输出:
1 2 3 4 5 6 7 8 9 10 11 12 13
| 可以跑。 -------------- 可以跑。 可以飞。 -------------- 可以跑。 可以游。 -------------- 可以跑。 可以飞。 可以游。 -------------- Hello world!
|