抽象工厂模式
设计模式
工厂模式只生产一个产品,而抽象工厂生产一个产品族。
角色及其职责
抽象工厂( Creator)角色
抽象工厂模式的核心,包合对多个产品结构的声明,任何工厂类都必须实现这个接口
具体工厂( Concrete Creator)角色
具体工厂类是抽象工厂的一个实现,负责实例化某个产品族中的产品对象。
抽象( Product)角色
抽象模式所创建的所有对象的父类,它负责描述所有实例所共有的公共接口。
具体产品( Concrete Product)角色
抽象模式所创建的具体实例对象。
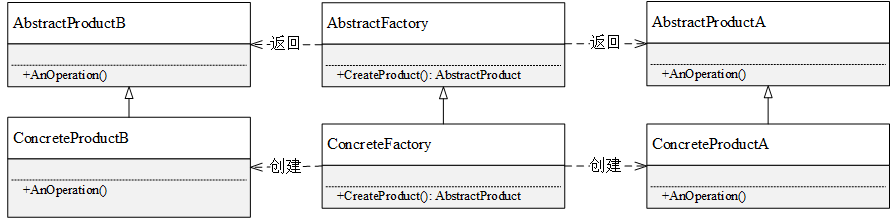
代码实现
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115
| #include <iostream> #include <string.h>
using namespace std;
class Fruit { public: virtual void getFruit() = 0; protected: private: };
class NorthBanana:public Fruit { public: virtual void getFruit() { cout << "get Northbanana!" << endl; } protected: private: };
class NorthApple:public Fruit { public: virtual void getFruit() { cout << "get Northapple!" << endl; } protected: private: };
class SourthBanana:public Fruit { public: virtual void getFruit() { cout << "get Sourthbanana!" << endl; } protected: private: };
class SourthApple:public Fruit { public: virtual void getFruit() { cout << "get Sourthapple!" << endl; } protected: private: };
class AbFactory { public: virtual Fruit* creatBanana() = 0; virtual Fruit* creatApple() = 0; protected: private: };
class NorthFactory:public AbFactory { public: virtual Fruit* creatBanana() { return new NorthBanana; } virtual Fruit* creatApple() { return new NorthApple; } protected: private: };
class SourthFactory:public AbFactory { public: virtual Fruit* creatBanana() { return new SourthBanana; } virtual Fruit* creatApple() { return new SourthApple; } protected: private: };
int main() { AbFactory *f = NULL; Fruit *fruit = NULL;
f = new NorthFactory; fruit = f->creatApple(); fruit->getFruit(); delete fruit; fruit = f->creatBanana(); fruit->getFruit(); delete fruit; delete f; cout << "Hello world!" << endl; return 0; }
|
输出:
1 2 3
| get Northapple! get Northbanana! Hello world!
|